Dealing with Nesting Hell: The Early Return Pattern
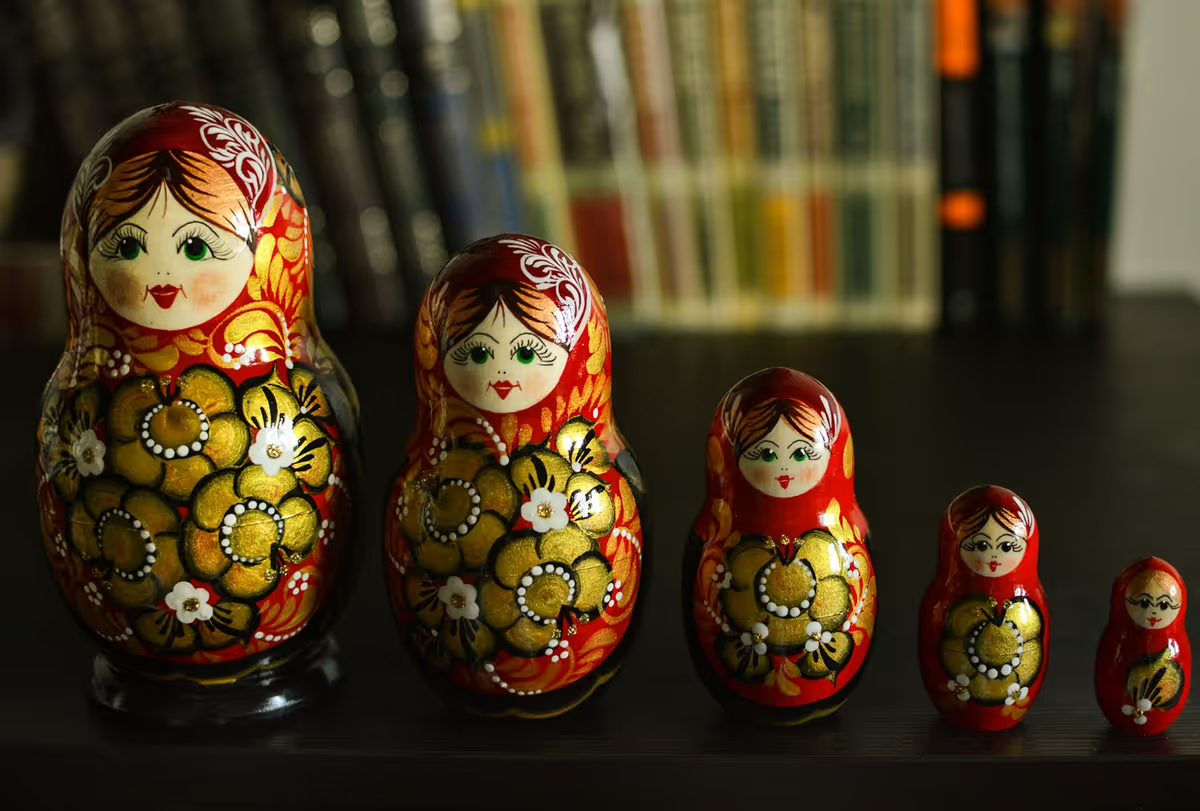
Image by @juliakadel on Unsplash
The problem
First, let's see a pseudo-function that fell into a problem of nesting hell.
./problematic.js
Copy
1/**2 * Pseudo fuction that demonstrates problem of not returning early3 */4function handleRequest(request) {5 if (request !== null && request !== undefined) {6 if (request.isValid) {7 if (request.user.isAuthenticated) {8 if (request.user.hasPermission("admin")) {9 // Process the request10 console.log("Doing some magic with yout request...");11 } else {12 console.log("User doesn't have permission.");13 }14 } else {15 console.log("User not authenticated.");16 }17 } else {18 console.log("Invalid request.");19 }20 } else {21 console.log("Request is missing.");22 }23}24
What problems can we identify?
- Code is not linear, multiple branching makes it much harder to analyze.
- All
else
statements are far away fromif
statements, and therefore it is hard to make connections between them. Even colorized brackets in the IDE don’t help. - It is very hard to refactor. If we want to cut a piece of logic and put it elsewhere, we might end up with dangling braces breaking our code or even worse, unexpected logical errors that come from hard-to-follow code.
Solution
The Early return pattern.
What is the Early Return Pattern?
The Early Return Pattern, as the name implies, is a way of writing functions/methods that return as soon as a condition allows it.
How to determine if code is not following this pattern?
- The
else
keyword
Usually, when theelse
keyword appears in our code, it means something is wrong. We should reconsider if using it is the right choice. - V-shaped functions
When your code's left side starts to form a V shape, it means that your code has a serious problem with excessive nesting. If, eventually, the length of your code grows to hundreds or even thousands of lines, you will quickly realize it is impossible to work with this code in a manageable way.
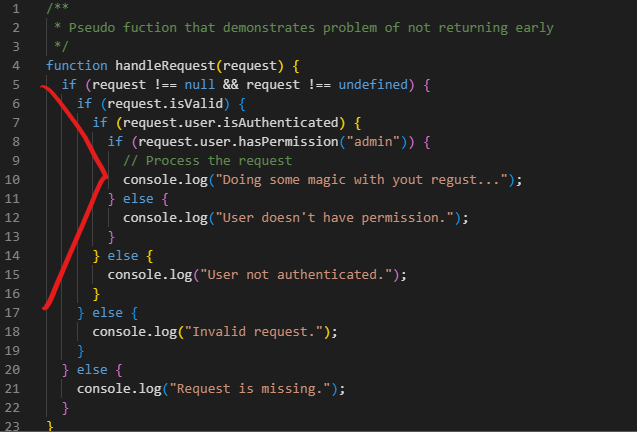
Example of V-shaped function
Time for fixing!
How do I refactor this code:
- Identify "Guard Clauses"
Find all conditions that validates function parameters, check tem as soon as possible and return or throw error if needed. - Identify branching in yout code
Whenever code execution splits between two blocks based on complex conditions (i.e., anif-else
pair), reduce it to a singleif
statement to prevent excessive nesting. Keep the "Happy Path" (the path expected to be executed most likely) at the function level, without nesting inside theif
.
./fixed.js
Copy
1/**2 * Pseudo fuction that demonstrates fixing the problem of not returning early3 */4function handleRequest(request) {5 // ================== Guard Clauses ==================6 if (request === null || request === undefined) {7 console.log("Request is missing.");8 return;9 }1011 if (!request.isValid) {12 console.log("Invalid request.");13 return;14 }15 // ================== Guard Clauses ==================1617 // Branching conditions flatened and splited in managable chunks18 if (!request.user.isAuthenticated) {19 console.log("User not authenticated.");20 return;21 }2223 // Branching conditions flatened and splited in managable chunks24 if (!request.user.hasPermission("admin")) {25 console.log("User doesn't have permission.");26 return;27 }2829 // Process the request if all checks pass30 console.log("Request processed.");31}32
And it's ready!
Summary
The Benefits of the Early Return Pattern:
- Easier to read code: Code is linear, and there is no hard-to-follow nesting.
- Easier to debug code: Each edge case can be easily debugged separately due to the absence of deep nesting.
- Easier to refactor: Simply cut off the code that you want and don't worry about gluing up braces.